Day14
Time : November 10th,2015 / Author : xiaomeixw
library
1.UI:
ProgressWheel --- (From Todd-Davies) : A progress wheel for android, intended for use instead of the standard progress bar. [loading加载].
SmoothProgressBar --- (From castorflex) : A small Android library allowing you to have a smooth and customizable horizontal indeterminate ProgressBar. [loading加载].
MaterialProgressBar --- (From DreaminginCodeZH) : Material design ProgressBar with consistent appearance. [Material design风格的loading加载].
MaterialLoadingProgressBar --- (From lsjwzh) : MaterialLoadingProgressBar provide a styled ProgressBar which looks like SwipeRefreshLayout's loading indicator(support-v4 v21+). [类谷歌SwipeRefreshLayout风格的loading加载].
android-square-progressbar --- (From mrwonderman) : An android library to display a progressbar that goes around an image. [图片loading提示].
GoogleProgressBar --- (From jpardogo) : Android library to display progress like google does in some of his services. [类谷歌风loading].
2.Logic:
jackdaw --- (From vbauer & Tag is Annotation) : Java Annotation Processor which allows to simplify development. [减少开发的常用注解关键词].
Jackdaw is a Java Annotation Processor which allows to simplify Java/Android development and prevents writing of tedious code.Jackdaw was inspired by Lombok project, but in comparison with Lombok:it does not need to have an extra plugin in IDE & it does not modify the existing source code.Jackdaw uses JitPack.io for distribution, so you need to configure JitPack's Maven repository to fetch artifacts (dependencies).It is necessary to make dependency on jackdaw-core. This module contains compile time annotations which will be used to give a hints for APT. Module jackdaw-apt contains annotation processor and all correlated logic.
**Jackdaw** supports the following compile time annotations:
<ul>
<li>@JAdapter</li>
<li>@JBean</li>
<li>@JBuilder</li>
<li>@JClassDescriptor</li>
<li>@JComparator</li>
<li>JFactoryMethod/li>
<li>@JFunction</li>
<li>@JMessage</li>
<li>@JPredicate</li>
<li>@JRepeatable</li>
<li>@JService</li>
<li>@JSupplier</li>
</ul>
<img src="http://i.imgur.com/WTt4hEb.png" width="90%">
EX: @JAdapter
//Original class MouseListener
@JAdapter
public interface MouseListener {
void onMove(int x, int y);
void onPressed(int button);
}
//Generated class MouseListenerAdapter
public class MouseListenerAdapter implements MouseListener {
public void onMove(final int x, final int y) {
}
public void onPresses(final int button) {
}
}
EX: @JBuilder
//Original class Company:
@JBuilder
public class Company {
private int id;
private String name;
private Set<String> descriptions;
public int getId() { return id; }
public void setId(final int id) { this.id = id; }
public String getName() { return name; }
public void setName(final String name) { this.name = name; }
public Set<String> getDescriptions() { return descriptions; }
public void setDescriptions(final Set<String> descriptions) { this.descriptions = descriptions; }
}
//Generated class CompanyBuilder:
public class CompanyBuilder {
private int id;
private String name;
private Set<String> descriptions;
public static CompanyBuilder create() {
return new CompanyBuilder();
}
public CompanyBuilder id(final int id) {
this.id = id;
return this;
}
public CompanyBuilder name(final String name) {
this.name = name;
return this;
}
public CompanyBuilder descriptions(final Set<String> descriptions) {
this.descriptions = descriptions;
return this;
}
public Company build() {
final Company object = new Company();
object.setId(id);
object.setName(name);
object.setListed(listed);
object.setDescriptions(descriptions);
return object;
}
}
3.Architecture:
Carpaccio --- (From florent37) : Data Mapping & Smarter Views framework for android. [各种View和Data绑定].
Data Mapping & Smarter Views framework for android.
With Carpaccio, your views became smarter, instead of calling functions on views, now your views can call functions ! You no longer need to extend a view to set a custom behavior
Carpaccio also come with a beautiful mapping engine !
##ImageViewController
```xml
<com.github.florent37.carpaccio.Carpaccio
app:register="
com.github.florent37.carpaccio.controllers.ImageViewController;
">
<ImageView
android:tag="
url(http://i.imgur.com/DvpvklR.png)
" />
</com.github.florent37.carpaccio.Carpaccio>
```
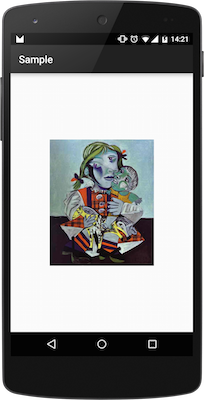
**WORKS WITH ANDROID STUDIO PREVIEW !!!**, don't hesitate to refresh your preview.
Preview an url image
```xml
<ImageView
android:tag="
enablePreview();
url(http://i.imgur.com/DvpvklR.png);
" />
```
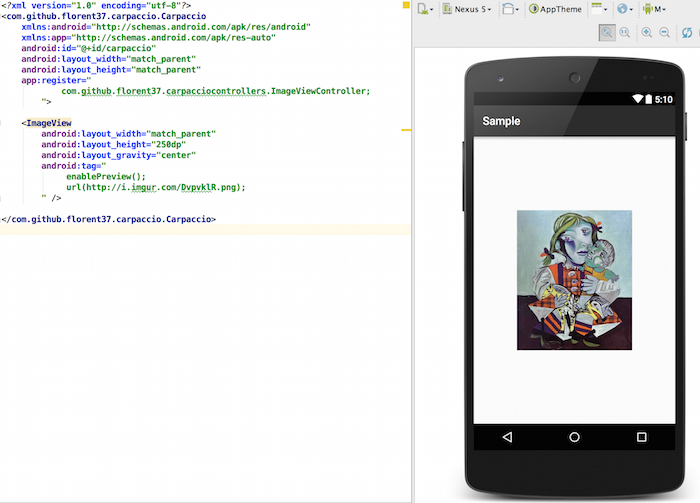
And some awesome customisations
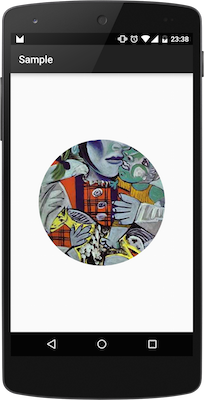
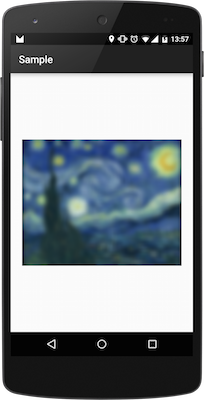
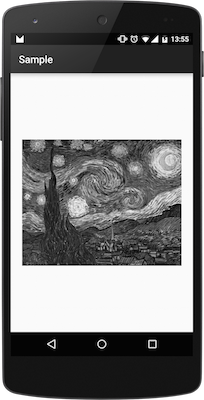
```xml
<ImageView
android:tag="
circle();
blur(); OR willBlur();
greyScale(); OR willGreyScale();
url(http://i.imgur.com/DvpvklR.png);
" />
```
[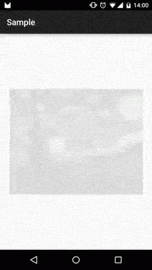](https://youtu.be/A0eyvpNh5wM)
[](https://youtu.be/4b84gswKGkA)
```xml
article
speed-up-your-app --- (From Author udinic blog http://blog.udinic.com/) --- [No Source]
A few weeks ago, I gave a talk about Android Performance Optimization, at Droidcon NYC. I invested a lot of time in this presentation, since I wanted to show real examples for performance issues, and how to identify them with the available tools. I had to cut down about a half of my slides because I didn’t have enough time to show everything. In this post, I’ll summarize everything I was talking about, and show examples I didn’t have time to go over..
My Rules
Everytime I approach a performance problem, or looking for performance problems, I follow these rules:
- _Always Measure - Optimizing with your eyes is never a good idea. After looking at the same animation for a few times, you’ll start imagining it’s running faster. Numbers don’t lie. Use the tools we’ll go over soon, and measure how your app performs a few times before and after you make your change._
- _Use slow devices - If you really want to expose all the weak spots, slower devices will help you more. Newer and stronger devices might not get too excited about performance issues you may have, but not all your users use the latest and greatest._
- _Trade-offs - Performance optimization is all about trade-offs. You optimize one thing - it comes on the expense of another. In many cases, that other thing can be your time spent finding and fixing it, but it can also be the quality of your bitmaps or the amount of data you should store in a specific data structure. Prepare yourself to make sacrifices._
[加速你的APP].
Chinese Translation Address : [Thanks To android-tech-frontier](http://www.devtf.cn/?p=1097)
<img src="http://i.imgur.com/cyGEUrC.png" width="90%">
<img src="http://i.imgur.com/FHdb7dt.png" width="90%">
<img src="http://i.imgur.com/x7N3sB9.png" width="90%">
